The following example will give you a clear explanation on
creating an Code First Entity Framework with sql server
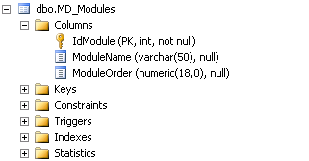
This is my Table, that has the
columns
1.IdModule
with PK and not null
2.ModuleName
with varchar and can be null
3.ModuleOrder
with numberic and can be null
These fields
can be changed in Entity Framework as
Field 1 : IdModule :
[Key]
[DatabaseGeneratedAttribute(DatabaseGeneratedOption.Identity)]
public int IdModule { get; set; }
where IdModule is
primary key and Identity automatically generated
Field 2 : ModuleName :
Field 2 : ModuleName :
public string ModuleName { get; set; }
Field 3 : ModuleOrder :
public decimal ModuleOrder { get; set; }
Now create one Model in
Models folder as the Name of the Table, here my table name is Md_Modules. So I
am creating one model as Md_Modules.cs
as
namespace MVC.Models
{
public class MD_Modules
{
[Key]
[DatabaseGeneratedAttribute(DatabaseGeneratedOption.Identity)]
public int IdModule { get; set; }
public string ModuleName { get; set; }
public decimal ModuleOrder { get; set; }
}
public class ModuleContext : DbContext
{
public ModuleContext()
: base("name=ApplicationDB")
{
}
public DbSet<MD_Modules> ModuleProfiles { get; set; }
}
}
Here I am created Context class as ModuleContext, you can give any name
Where ModuleContext is used for connecting with Database.
Here we need to pass the
string name=ApplicationDB to
the DbContext using Base Constructor
in ModuleContext where the Parameter string is the
name of connection string, Here my connection string name is ApplicationDB, so
I am passing the parameter as name=ApplicationDB.
<connectionStrings>
<add
name="ApplicationDB"
connectionString="server=192.168.0.7;database=AdeptaInternal;uid=AdeptaInternal;pwd=welcome@123;" providerName="System.Data.SqlClient"/>
</connectionStrings>
Then add a Controller as right click on Controller and Select Add Controller and Click on Ok
then you can find the following screen
1.Give the Controller Name
2.Selct MVC controller with read/write actions and views, using Entity Framework from the template dropdowm.
3.Select Model class
4.Select Data Context Class
5.Click on Add .
Once you click on Add , following code is generated automatically in your added controller.
While executing your solution control will check for controller in route config file
by default controller is Home and action is Index.So controll start from Index method in Home control.
Here Control comes to Index method. here we are returning the entire data in Md_Module table ( class).
Then add a Controller as right click on Controller and Select Add Controller and Click on Ok
then you can find the following screen
1.Give the Controller Name
2.Selct MVC controller with read/write actions and views, using Entity Framework from the template dropdowm.
3.Select Model class
4.Select Data Context Class
5.Click on Add .
Once you click on Add , following code is generated automatically in your added controller.
namespace MVCLera.Controllers
{
public class Default1Controller : Controller
{
private ModuleContext db = new ModuleContext();
//
// GET: /Default1/
public ActionResult Index()
{
return View(db.ModuleProfiles.ToList());
}
While executing your solution control will check for controller in route config file
by default controller is Home and action is Index.So controll start from Index method in Home control.
Here Control comes to Index method. here we are returning the entire data in Md_Module table ( class).
public ActionResult Details(int? id = null)
{
MD_Modules md_modules = db.ModuleProfiles.Find(id);
if (md_modules == null)
{
return HttpNotFound();
}
return View(md_modules);
}
//
// GET: /Default1/Create
public ActionResult Create()
{
return View();
}
//
// POST: /Default1/Create
[HttpPost]
public ActionResult Create(MD_Modules md_modules)
{
if (ModelState.IsValid)
{
db.ModuleProfiles.Add(md_modules);
db.SaveChanges();
return RedirectToAction("Index");
}
return View(md_modules);
}
//
// GET: /Default1/Edit/5
public ActionResult Edit(int? id = 0)
{
MD_Modules md_modules = db.ModuleProfiles.Find(id);
if (md_modules == null)
{
return HttpNotFound();
}
return View(md_modules);
}
//
// POST: /Default1/Edit/5
[HttpPost]
public ActionResult Edit(MD_Modules md_modules)
{
if (ModelState.IsValid)
{
db.Entry(md_modules).State = EntityState.Modified;
db.SaveChanges();
return RedirectToAction("Index");
}
return View(md_modules);
}
//
// GET: /Default1/Delete/5
public ActionResult Delete(int? id = null)
{
MD_Modules md_modules = db.ModuleProfiles.Find(id);
if (md_modules == null)
{
return HttpNotFound();
}
return View(md_modules);
}
//
// POST: /Default1/Delete/5
[HttpPost, ActionName("Delete")]
public ActionResult DeleteConfirmed(int? id)
{
MD_Modules md_modules = db.ModuleProfiles.Find(id);
db.ModuleProfiles.Remove(md_modules);
db.SaveChanges();
return RedirectToAction("Index");
}
protected override void Dispose(bool disposing)
{
db.Dispose();
base.Dispose(disposing);
}
}
}
No comments:
Post a Comment